Job Sequencing Problem Statement
You are provided with a N x 2 2-D array called Jobs
consisting of N
jobs. In this array, Jobs[i][0]
represents the deadline of the i-th job, while Jobs[i][1]
indicates the profit linked with the i-th job.
Your objective is to determine the maximum profit that can be attained by scheduling jobs such that each job is completed within its respective deadline. Note that every job requires one unit of time to complete, and only one job can be scheduled at a particular time.
Example:
Input:
jobs = [[2, 40], [2, 20], [1, 10]]
Output:
60
Explanation:
In this example, it's optimal to complete the first and second jobs to achieve a profit of 60. The first job can be completed in the first unit of time and the second job in the second unit. Thus, the total profit is 40 + 20 = 60
. The third job cannot be completed within its deadline, thus no profit is earned from it.
Constraints:
1 ≤ T ≤ 100
1 ≤ N ≤ 5000
1 ≤ jobs[i][0] ≤ 3000
1 ≤ jobs[i][1] ≤ 105
- Time limit: 1 sec
Note:
If a job has a deadline 'x', it must be completed at any time before 'x'. You only need to implement the function, without handling input and output, which is already managed.
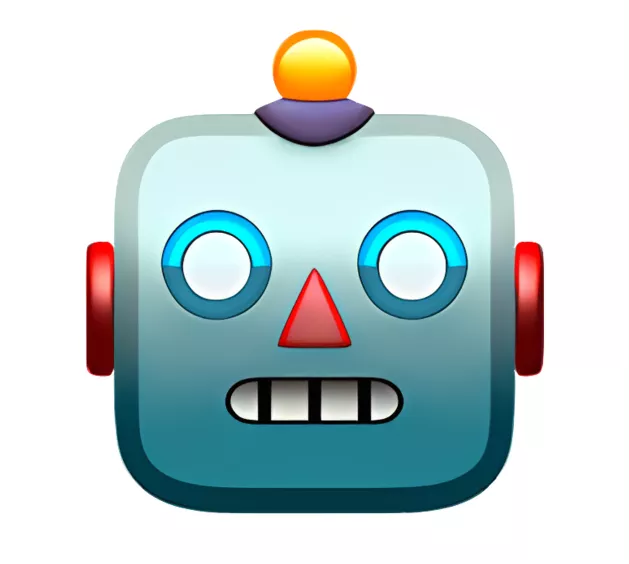
The objective is to determine the maximum profit by scheduling jobs within their deadlines.
Sort the jobs array based on the profit in descending order.
Iterate through the sorted array and schedule job...read more
NCR Corporation Associate Software Engineer interview questions & answers
Popular interview questions of Associate Software Engineer
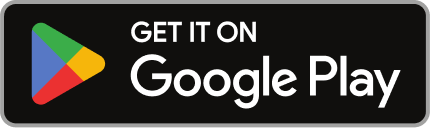
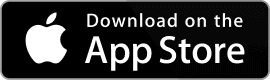
Reviews
Interviews
Salaries
Users
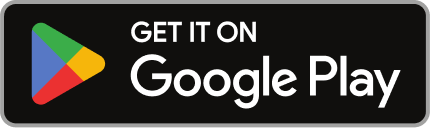
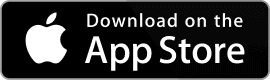