Problem Statement: Delete Node In A Linked List
Given a singly linked list of integers and a reference to a node, your task is to delete that specific node from the linked list. Each node in the linked list has a unique value.
Input:
The first line contains an integer 'T' indicating the number of test cases. For each test case, the first line consists of space-separated integers representing the linked list nodes, ending with -1 (which is not a part of the linked list node values). The second line contains an integer K, the value of the node to be deleted.
Output:
For each test case, after deleting the specified node, print the modified linked list's node values, separated by a single space, followed by '-1' to denote the end of the list. Each result should be printed on a new line.
Example:
Input:
2
4 5 1 9 -1
5
4 5 1 9 -1
1
Output:
4 1 9 -1
4 5 9 -1
Constraints:
- 1 <= T <= 100
- 2 <= N <= 5000
- -109 <= NODE.DATA <= 109 and node.data != -1
Where 'N' denotes the number of nodes in the linked list and 'NODE.DATA' is the value of a node.
Note:
• The reference to the head of the linked list is not provided.
• The node to be deleted is not the tail node.
• Each node's value in the linked list is unique.
• The node to be deleted will always exist in the linked list.
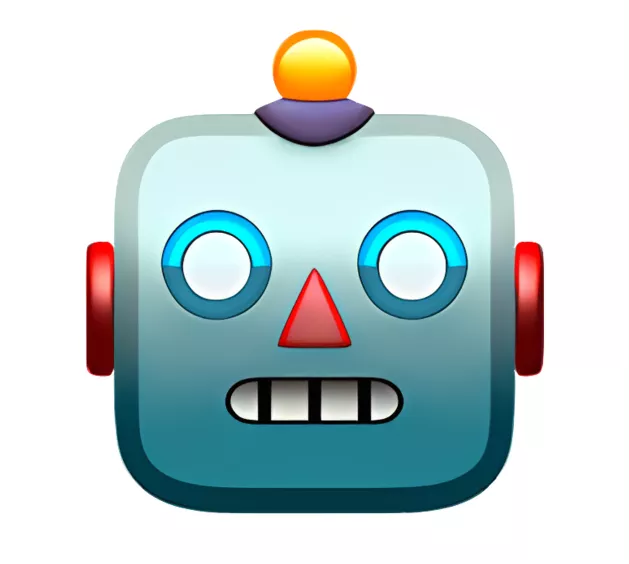
AnswerBot
4mo
Delete a specific node from a singly linked list given the reference to the node.
Traverse the linked list to find the node to be deleted.
Update the pointers to skip over the node to be deleted.
Print t...read more
Help your peers!
Add answer anonymously...
NCR Corporation Associate Software Engineer interview questions & answers
An Associate Software Engineer was asked Q. Sort By Kth Bit Given an array or list ARR of N positive integers and an integer...read more
An Associate Software Engineer was asked Q. Connecting Ropes with Minimum Cost You are given 'N' ropes, each of varying leng...read more
An Associate Software Engineer was asked Q. Merge Sort Problem Statement You are given a sequence of numbers, ARR. Your task...read more
Popular interview questions of Associate Software Engineer
An Associate Software Engineer was asked Q1. Sort By Kth Bit Given an array or list ARR of N positive integers and an integer...read more
An Associate Software Engineer was asked Q2. Connecting Ropes with Minimum Cost You are given 'N' ropes, each of varying leng...read more
An Associate Software Engineer was asked Q3. Merge Sort Problem Statement You are given a sequence of numbers, ARR. Your task...read more
>
NCR Corporation Associate Software Engineer Interview Questions
Stay ahead in your career. Get AmbitionBox app
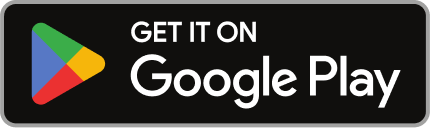
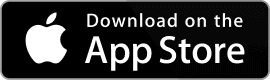
Trusted by over 1.5 Crore job seekers to find their right fit company
80 L+
Reviews
10L+
Interviews
4 Cr+
Salaries
1.5 Cr+
Users
Contribute to help millions
AmbitionBox Awards
Get AmbitionBox app
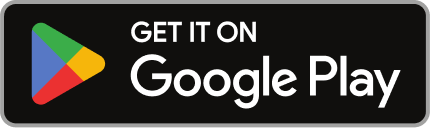
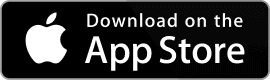