Word Break Problem Statement
You are given a list of N
strings called A
. Your task is to determine whether you can form a given target string by combining one or more strings from A
.
The strings from A
can be used multiple times to form the target.
Example:
Input:
A = ["coding", "ninjas", "is", "awesome"]
target = "codingninjas"
Output:
true
Explanation:
We can use the strings "coding" and "ninjas" to form the target "codingninjas".
Input:
The first line of input contains a single integer T, representing the number of test cases or queries to be run.
Then the T test cases follow.
The first line of each test contains a single integer N denoting the total number of strings in A.
The second line of each test contains "N" space-separated strings of A.
The third line of each test contains a single string target.
Output:
For each test case, print 1 if you can form a target string, otherwise print 0.
The output of each test case will be printed in a separate line.
Constraints:
- 1 <= T <= 5
- 1 <= N, | target | <= 102
- 1 <= | A[i] | <= 10
- Strings in A contain only lowercase English characters.
Note:
You do not need to print anything; it has already been handled. Just implement the given function.
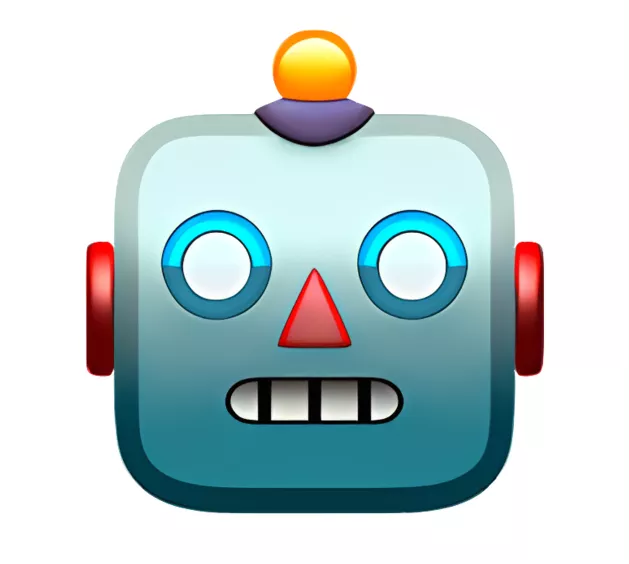
AnswerBot
4mo
Given a list of strings, determine if a target string can be formed by combining one or more strings from the list.
Iterate through all possible combinations of strings from the list to check if they c...read more
Help your peers!
Add answer anonymously...
Nagarro Software Developer Intern interview questions & answers
A Software Developer Intern was asked Q. Word Break Problem Statement You are given a list of N strings called A. Your ta...read more
A Software Developer Intern was asked Q. Sort 0 and 1 Problem Statement Given an integer array ARR of size N containing o...read more
A Software Developer Intern was asked Q. Check If Binary Representation of a Number is Palindrome Given an integer N, det...read more
Popular interview questions of Software Developer Intern
A Software Developer Intern was asked Q1. Word Break Problem Statement You are given a list of N strings called A. Your ta...read more
A Software Developer Intern was asked Q2. Sort 0 and 1 Problem Statement Given an integer array ARR of size N containing o...read more
A Software Developer Intern was asked Q3. Check If Binary Representation of a Number is Palindrome Given an integer N, det...read more
Stay ahead in your career. Get AmbitionBox app
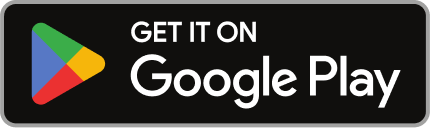
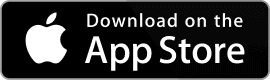
Trusted by over 1.5 Crore job seekers to find their right fit company
80 L+
Reviews
10L+
Interviews
4 Cr+
Salaries
1.5 Cr+
Users
Contribute to help millions
AmbitionBox Awards
Get AmbitionBox app
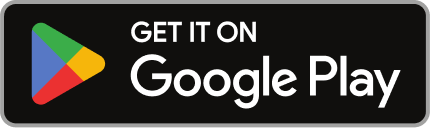
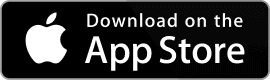