Day of the Week Calculation
Your task is to create a function that determines the day of the week for any given date, whether in the past or the future.
Input:
The first line consists of an integer 'T', representing the number of queries. Each query line contains three integers: 'Day', 'Month', and 'Year'.
Output:
For each query, output the corresponding day of the week as a single string: {"Sunday", "Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday"}.
Example:
For the input date 28 8 2020
, the output should be Friday
because 28th August 2020 is a Friday.
Constraints:
1 <= T <= 10^5
1 <= Day <= 31
1 <= Month <= 12
1 <= Year <= 2,000,000
Time Limit: 1 second.
Note:
The input is guaranteed to be a valid date.
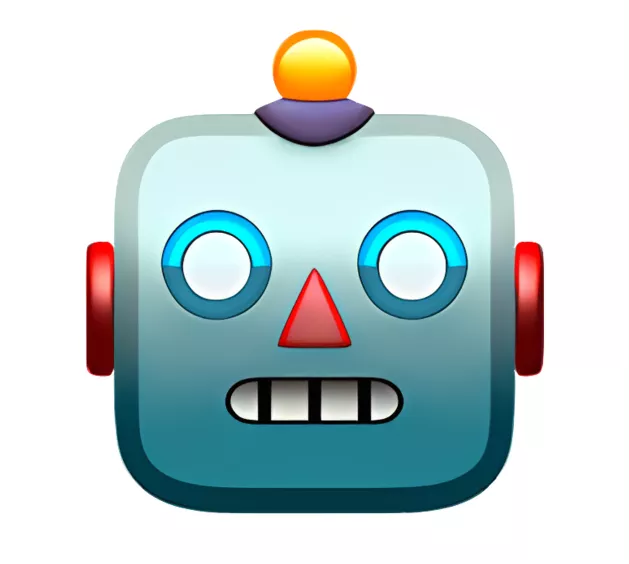
AnswerBot
4mo
Create a function to determine the day of the week for any given date.
Parse the input integers to create a date object
Use a library or algorithm to calculate the day of the week for the given date
Retu...read more
Help your peers!
Add answer anonymously...
Microsoft Corporation Software Developer interview questions & answers
A Software Developer was asked 9mo agoQ. Given an m x n 2D binary grid grid which represents a map of '1's (land) and '0'...read more
A Software Developer was asked 10mo agoQ. What is your long-term goal?
A Software Developer was asked 11mo agoQ. Tell me about graphs.
Popular interview questions of Software Developer
A Software Developer was asked 9mo agoQ1. Given an m x n 2D binary grid grid which represents a map of '1's (land) and '0'...read more
A Software Developer was asked 10mo agoQ2. What is your long-term goal?
A Software Developer was asked 11mo agoQ3. Tell me about graphs.
>
Microsoft Corporation Software Developer Interview Questions
Stay ahead in your career. Get AmbitionBox app
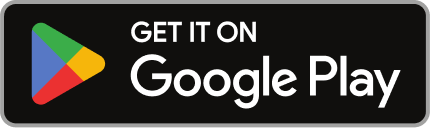
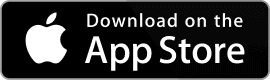
Trusted by over 1.5 Crore job seekers to find their right fit company
80 L+
Reviews
10L+
Interviews
4 Cr+
Salaries
1.5 Cr+
Users
Contribute to help millions
AmbitionBox Awards
Get AmbitionBox app
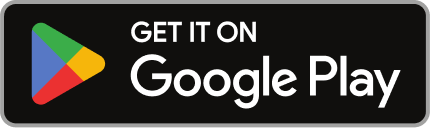
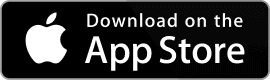