Problem: Count Even or Odd in Array
Tanmay and Rohit are best buddies. Tanmay gives Rohit a challenge involving an array of N natural numbers. The task is to perform and answer a series of queries on the array as follows:
Explanation:
Query Types:- Query 0:
0 x y
- Update the element at indexx
toy
in the array. - Query 1:
1 x y
- Count the number of even numbers in the range from indexx
toy
(inclusive). - Query 2:
2 x y
- Count the number of odd numbers in the range from indexx
toy
(inclusive).
Input:
The first line contains an integer 'N', the size of the array 'arr'.
Next line contains 'N' space-separated integers representing 'arr'.
The following line contains an integer 'q', the number of queries.
The next 'q' lines contain one of the specified queries (0 x y, 1 x y, or 2 x y).
Output:
For each query of type 1 and 2, output the result on a new line.
Example:
Input:
6
1 3 5 7 9 2
5
1 0 4
0 3 6
1 0 4
2 0 5
2 2 4
Output:
0
0
6
2
Constraints:
- 1 ≤ N, Q ≤ 105
- 0 ≤ x ≤ y ≤ N - 1
- 0 ≤ arr[i] ≤ 109
- 1 ≤ x ≤ N
- 0 ≤ y ≤ 109
Note:
No need to print any output yourself, implement the function as specified.
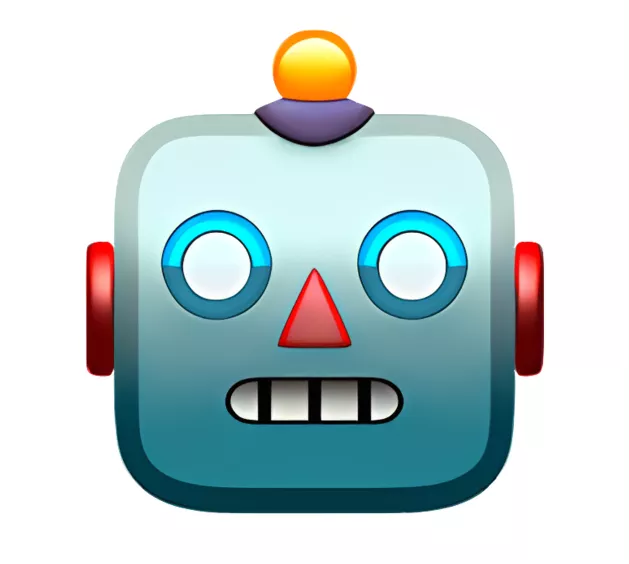
AnswerBot
4mo
Count the number of even or odd numbers in a range of an array based on given queries.
Create an array to store the input numbers.
Iterate through the queries and update or count even/odd numbers based ...read more
Help your peers!
Add answer anonymously...
LTIMindtree Software Developer interview questions & answers
A Software Developer was asked 1mo agoQ. What is a Common Table Expression (CTE) in SQL?
A Software Developer was asked 2mo agoQ. Given a string, how would you count the occurrences of each character?
A Software Developer was asked 6mo agoQ. Write the project code
Popular interview questions of Software Developer
A Software Developer was asked 3w agoQ1. What is a Common Table Expression (CTE) in SQL?
A Software Developer was asked 2mo agoQ2. Given a string, how would you count the occurrences of each character?
A Software Developer was asked 6mo agoQ3. Write the project code
Stay ahead in your career. Get AmbitionBox app
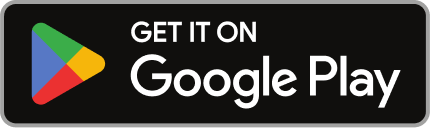
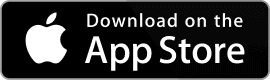
Trusted by over 1.5 Crore job seekers to find their right fit company
80 L+
Reviews
10L+
Interviews
4 Cr+
Salaries
1.5 Cr+
Users
Contribute to help millions
AmbitionBox Awards
Get AmbitionBox app
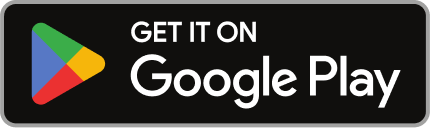
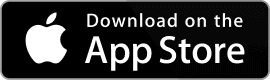