K Closest Points to Origin Problem Statement
Your house is located at the origin (0,0) of a 2-D plane. There are N
neighbors living at different points on the plane. Your goal is to visit exactly K
neighbors who are closest to your house. Given a 2-D list POINTS
, find out the coordinates of the neighbors you will visit. The result will be unique.
Note:
The distance between two points on a plane is calculated using the Euclidean Distance.
Example:
Input:
N = 2, K = 1, POINTS = [[2, 3], [-1, 2]]
Output:
[[-1, 2]]
Explanation:
The distance of the first point (2,3) from the origin is √((2)^2 + (3)^2) = √13. The distance of the second point (-1,2) from the origin is √((-1)^2 + (2)^2) = √5. The closest point is [-1, 2].
Constraints:
1 ≤ T ≤ 10
1 ≤ K ≤ N ≤ 10^4
-10^4 ≤ POINTS[i][0], POINTS[i][1] ≤ 10^4
- Time limit: 1 sec.
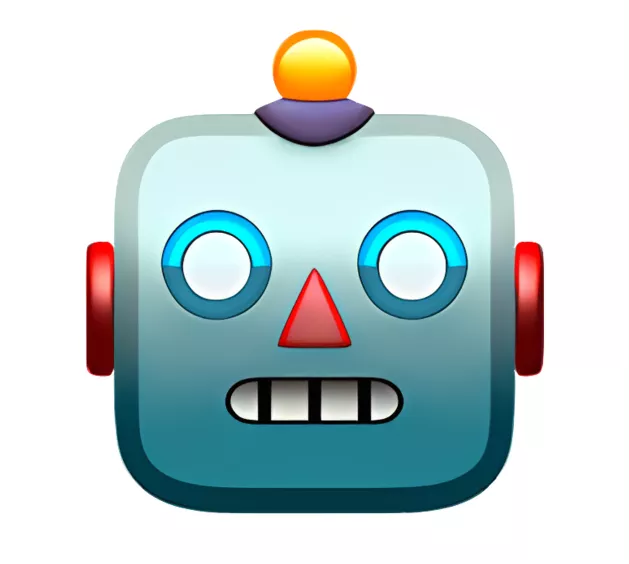
Find the K closest points to the origin in a 2-D plane using Euclidean Distance.
Calculate the Euclidean Distance of each point from the origin
Sort the points based on their distances from the origin
Re...read more
Jio Software Developer interview questions & answers
Popular interview questions of Software Developer
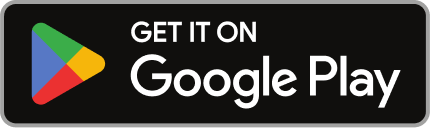
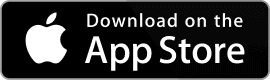
Reviews
Interviews
Salaries
Users
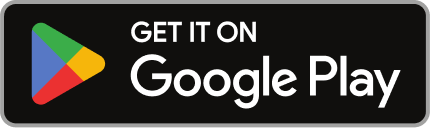
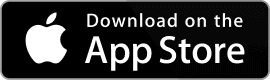