Problem Statement: Check Whether Binary Tree Is Complete
You are provided with a binary tree. Your task is to determine if the given binary tree is a Complete Binary Tree.
A Complete Binary Tree is defined as a binary tree in which every level, except possibly the last, is entirely filled, and all nodes in the final level are positioned to the left.
Input:
The input begins with an integer T
, indicating the number of test cases. For each test case, a sequence of node values is given in level order traversal, where the values are separated by spaces. If a node is null, it is represented by -1
.
1
2 3
4 -1 5 6
-1 7 -1 -1 -1 -1
-1 -1
Output:
For each test case, output 1
if the Binary Tree is complete, and 0
if it is not. Continuous lines indicate individual test case outputs.
Example:
Input:
1 2 3 4 -1 5 6 -1 7 -1 -1 -1 -1 -1 -1
Output:
1
Constraints:
1 ≤ T ≤ 100
1 ≤ N ≤ 3000
, whereN
is the total number of nodes in the binary tree.1 ≤ data ≤ 105
anddata ≠ -1
Note:
No need to print anything for solving the problem; the implementation should return the results directly.
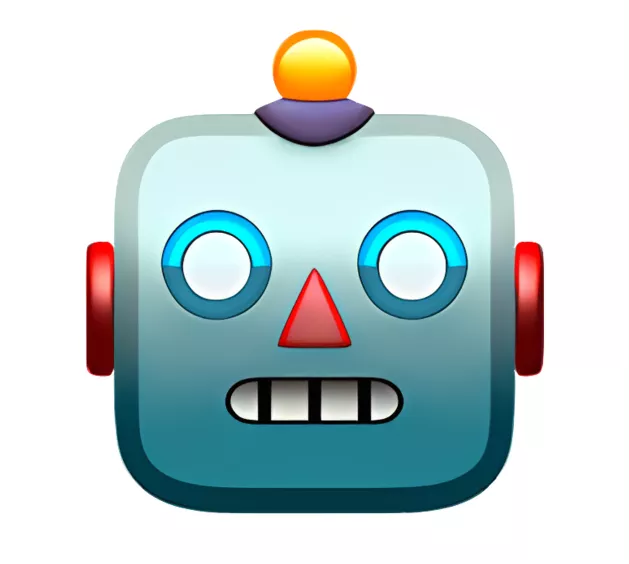
The task is to check whether a given binary tree is a complete binary tree or not.
A complete binary tree is a binary tree where every level, except possibly the last, is completely filled.
All nodes in...read more
Jio Software Developer interview questions & answers
Popular interview questions of Software Developer
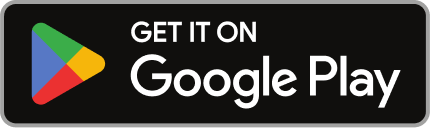
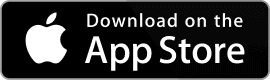
Reviews
Interviews
Salaries
Users
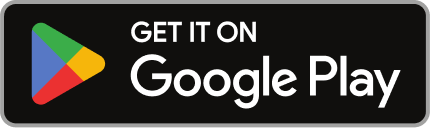
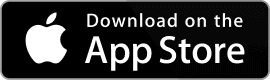