Write a Java program using String with collections.
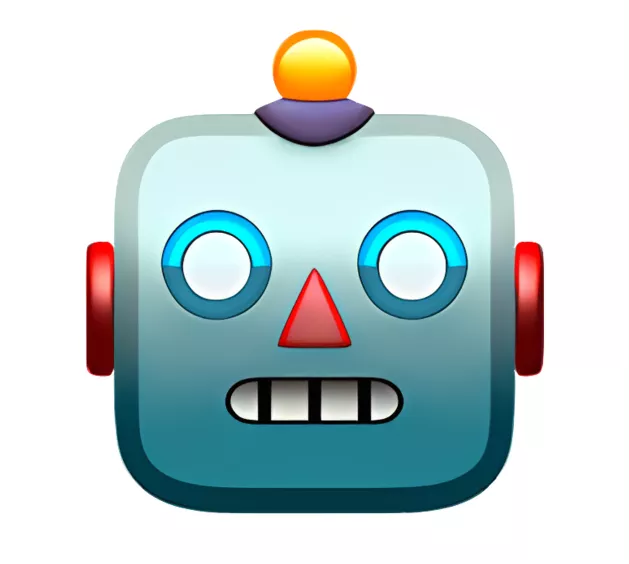
Java collections can store arrays of strings
Use ArrayList or HashSet to store arrays of strings
ArrayList allows duplicates while HashSet does not
Example: ArrayList<String> names = new ArrayList<>();
Ex...read more
package com.java;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
public class String_Collection {
public static void main(String[] args) {
// List example
List<String> stringList = new ArrayList<>();
stringList.add("apple");
stringList.add("banana");
stringList.add("orange");
System.out.println("List Example:");
for (String fruit : stringList) {
System.out.println(fruit);
}
// Set example
Set<String> stringSet = new HashSet<>();
stringSet.add("apple");
stringSet.add("banana");
stringSet.add("orange");
System.out.println("\nSet Example:");
for (String fruit : stringSet) {
System.out.println(fruit);
}
// Map example
Map<Integer, String> stringMap = new HashMap<>();
stringMap.put(1, "apple");
stringMap.put(2, "banana");
stringMap.put(3, "orange");
System.out.println("\nMap Example:");
for (Map.Entry<Integer, String> entry : stringMap.entrySet()) {
System.out.println(entry.getKey() + ": " + entry.getValue());
}
}
}
In this example, we've used three different Java collections: ArrayList
(for a list of strings), HashSet
(for a set of strings), and HashMap
(for a map with integer keys and string values). The program adds strings to each collection and then demonstrates iterating through and printing the elements
IBM Specialist Testing interview questions & answers
Popular interview questions of Specialist Testing
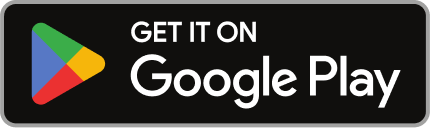
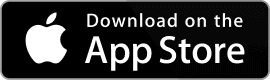
Reviews
Interviews
Salaries
Users
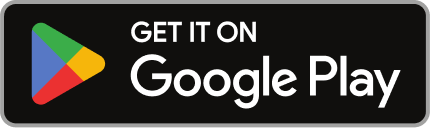
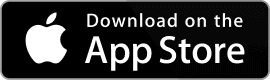