Dijkstra's Shortest Path Problem
Given an undirected graph with ‘V’ vertices (labeled 0, 1, ... , V-1) and ‘E’ edges, where each edge has a weight representing the distance between two connected nodes (X, Y).
You need to determine the shortest path distance from the source node (labeled as 0) to all vertices in the graph.
Input:
The first line contains an integer 'T' representing the number of test cases.
Each test case starts with a line containing two integers 'V' and 'E' indicating the number of vertices and edges, respectively.
The subsequent ‘E’ lines each contain three space-separated integers, 'X', 'Y', and 'DISTANCE', describing an edge between nodes 'X' and 'Y' with a specified distance.
Output:
For each test case, output a single line with ‘V’ space-separated integers representing the shortest distances from the source node 0 to all other nodes. For a disconnected vertex, use the integer value 2147483647.
Example:
Input:
4 5
0 1 5
0 2 8
1 2 9
1 3 2
2 3 6
Output:
0 5 8 7
Explanation:
In the provided input, there are 4 vertices and 5 edges. The edge list indicates:
- An edge between node 0 and node 1 with a distance of 5
- An edge between node 0 and node 2 with a distance of 8
- An edge between node 1 and node 2 with a distance of 9
- An edge between node 1 and node 3 with a distance of 2
- An edge between node 2 and node 3 with a distance of 6
The output shows the shortest path distances from node 0 to all other nodes: 0, 5, 8, 7.
Constraints:
1 ≤ T ≤ 50
1 ≤ V ≤ 1000
1 ≤ E ≤ 3000
0 ≤ X, Y < V
1 ≤ DISTANCE[X][Y] ≤ 105
Note:
- No self-loops are present in the graph.
- Parallel edges may exist, meaning two nodes can have more than one edge between them.
- You do not need to print the output; focus on implementing the solution function.
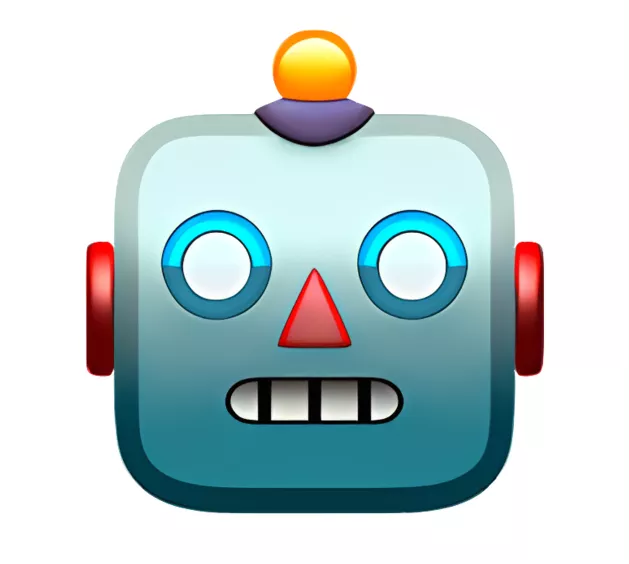
Dijkstra's algorithm is used to find the shortest path from a source node to all other nodes in a graph with weighted edges.
Implement Dijkstra's algorithm to find the shortest path distances from the ...read more
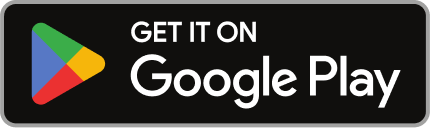
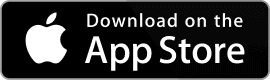
Reviews
Interviews
Salaries
Users
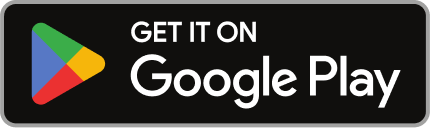
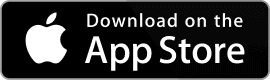