Shortest Alternate Colored Path Problem
Given a directed graph consisting of 'N' nodes labeled from '0' to 'N-1'. Each edge in the graph is colored either 'red' or 'blue'. The graph may include self-edges and parallel edges. You have two arrays, redEdges
and blueEdges
, each containing edges in the form [i, j], representing an edge from node 'i' to node 'j' with the respective color.
The task is to compute an array answer
of size 'N', where answer[i]
denotes the length of the shortest path from node '0' to node 'i' with alternating colored edges. If no such path exists, answer[i]
should be -1.
Input:
The first line contains an integer ‘T’ representing the number of test cases. Each test case begins with three space-separated integers 'N', 'rlen', and 'blen', indicating the number of nodes, and the sizes of the arrays redEdges
and blueEdges
respectively.
The following rlen
lines each contain two space-separated integers 'i' and 'j', indicating a 'red' edge.
The following blen
lines each contain two space-separated integers 'i' and 'j', indicating a 'blue' edge.
Output:
For each test case, output a single line containing 'N' space-separated integers, representing the answer
array with the shortest path lengths from node '0' to each node.
Example:
Input:
N = 4, redEdges = [[0, 1], [2, 3]], blueEdges = [[1, 2], [1, 3]]
Output:
[0, 1, 2, 2]
Explanation:
The shortest paths from node ‘0’ are:
Node 1: 0->1, Length: 1
Node 2: 0->1->2, Length: 2
Node 3: 0->1->3, Length: 2
Hence, the result array is [0, 1, 2, 2].
Constraints:
1 ≤ T ≤ 100
1 ≤ N ≤ 200
0 ≤ rlen + blen ≤ 1000
Note:
The graph can be disconnected, and there may only be one red or one blue edge between any two distinct nodes. Implementation should only return the results as output is handled separately.
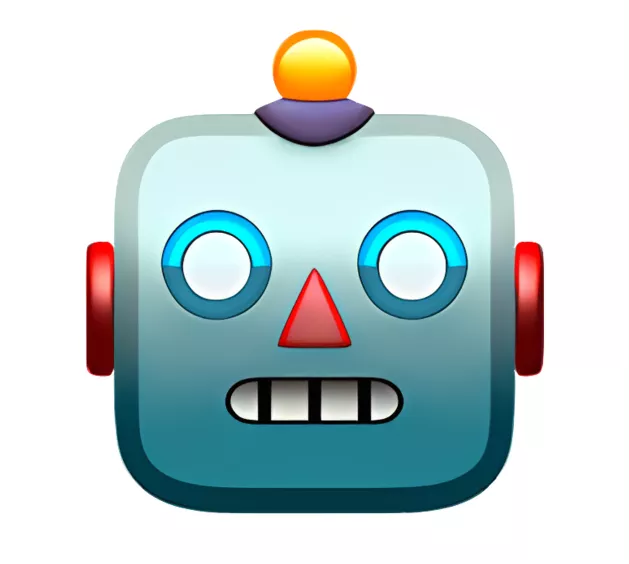
The task is to compute the shortest path from node '0' to each node in a directed graph with alternating colored edges.
Create a graph using the given red and blue edges.
Use Breadth First Search (BFS) ...read more
Flipkart Software Developer interview questions & answers
Popular interview questions of Software Developer
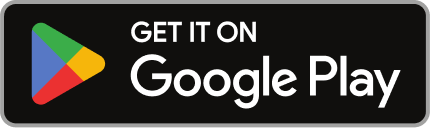
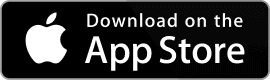
Reviews
Interviews
Salaries
Users
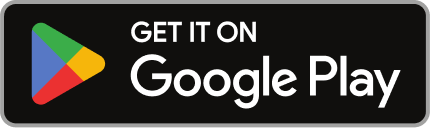
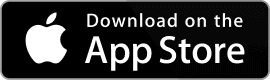