Binary Tree to Doubly Linked List
Transform a given Binary Tree into a Doubly Linked List.
Ensure that the nodes in the Doubly Linked List follow the Inorder Traversal of the Binary Tree.
Input:
The first line contains an integer 'T' representing the number of test cases. For each test case, provide the tree nodes in level order as a single space-separated string of integers. Use -1 to denote null nodes.
Output:
For each test case, return the head of the Doubly Linked List.
Example:
Input:
1
1 2 3 4 -1 5 6 -1 7 -1 -1 -1 -1 -1 -1
Output:
The head to the doubly linked list with elements in order: 4 -> 2 -> 7 -> 1 -> 5 -> 3 -> 6
Constraints:
- 1 ≤ T ≤ 100
- 0 ≤ N ≤ 3000
- -10^6 ≤ data ≤ 10^6 and data ≠ -1
Note:
Output is handled internally. Implement the function to perform the conversion.
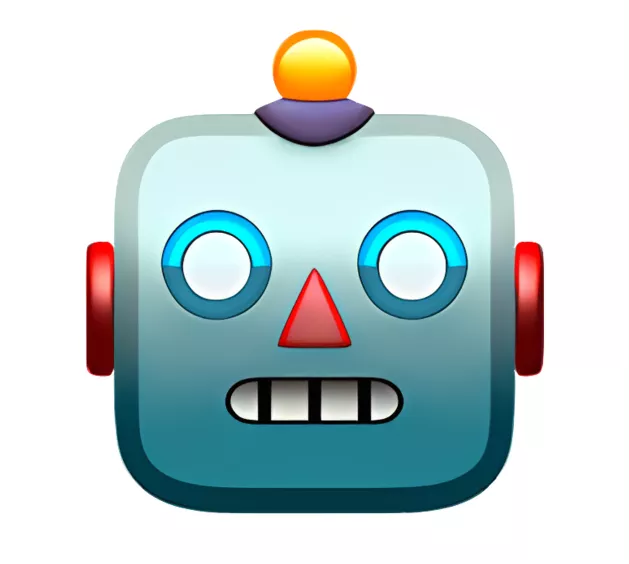
AnswerBot
4mo
Convert a Binary Tree into a Doubly Linked List following Inorder Traversal.
Perform Inorder Traversal of the Binary Tree to get the nodes in order.
Create a Doubly Linked List by connecting the nodes i...read more
Help your peers!
Add answer anonymously...
Expedia Group Software Developer interview questions & answers
A Software Developer was asked Q. Knight Probability in Chessboard Calculate the probability that a knight remains...read more
A Software Developer was asked Q. Remove Character from String Problem Statement Given a string str and a characte...read more
A Software Developer was asked Q. Binary Tree to Doubly Linked List Transform a given Binary Tree into a Doubly Li...read more
Popular interview questions of Software Developer
A Software Developer was asked Q1. Knight Probability in Chessboard Calculate the probability that a knight remains...read more
A Software Developer was asked Q2. Remove Character from String Problem Statement Given a string str and a characte...read more
A Software Developer was asked Q3. Binary Tree to Doubly Linked List Transform a given Binary Tree into a Doubly Li...read more
>
Expedia Group Software Developer Interview Questions
Stay ahead in your career. Get AmbitionBox app
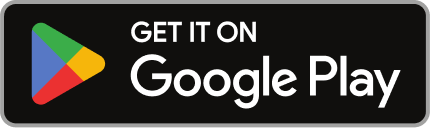
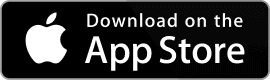
Trusted by over 1.5 Crore job seekers to find their right fit company
80 L+
Reviews
10L+
Interviews
4 Cr+
Salaries
1.5 Cr+
Users
Contribute to help millions
AmbitionBox Awards
Get AmbitionBox app
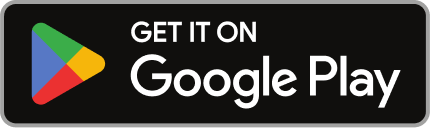
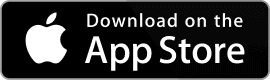