Palindrome Partitioning Problem Statement
You are given a string S
. Your task is to partition S
such that every substring of the partition is a palindrome. Your objective is to return all possible palindrome partitioning configurations for S
.
Example:
Input:
"BaaB"
Output:
["B", "a", "a", "B"]
["B", "aa", "B"]
["BaaB"]
Explanation:
There are three possible palindrome partitioning of the string "BaaB":
- ["B", "a", "a", "B"]
- ["B", "aa", "B"]
- ["BaaB"]
In each partition, every substring is a palindrome.
Constraints:
- 0 <= |S| <= 15
- |S| denotes the length of string
S
. - Time Limit: 1 sec.
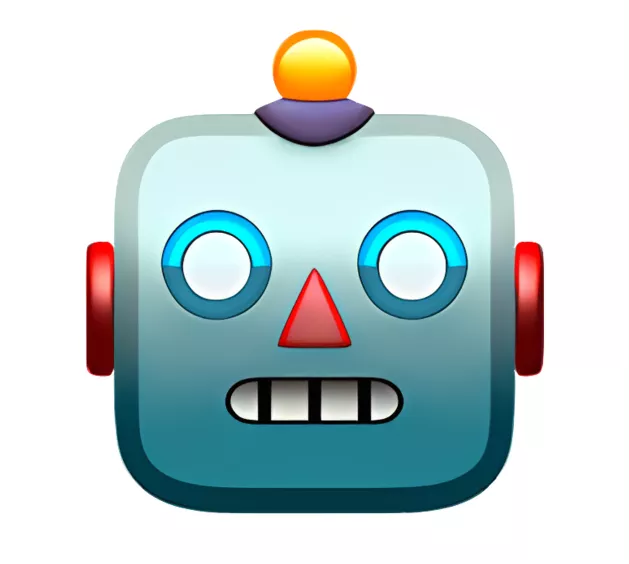
AnswerBot
4mo
Given a string, partition it into palindromes and return all possible configurations.
Use backtracking to generate all possible palindrome partitions
Check if each substring is a palindrome before addin...read more
Help your peers!
Add answer anonymously...
Amazon Software Developer Intern interview questions & answers
A Software Developer Intern was asked 4mo agoQ. Given a linked list, find the n'th node from the end of the list.
A Software Developer Intern was asked 4mo agoQ. Given a tree, find its diameter (the longest path between two nodes in the tree)...read more
A Software Developer Intern was asked 4mo agoQ. Given a matrix, find the shortest distance between two given points located anyw...read more
Popular interview questions of Software Developer Intern
A Software Developer Intern was asked 4mo agoQ1. Given a linked list, find the n'th node from the end of the list.
A Software Developer Intern was asked 4mo agoQ2. Given a tree, find its diameter (the longest path between two nodes in the tree)...read more
A Software Developer Intern was asked 4mo agoQ3. Given a matrix, find the shortest distance between two given points located anyw...read more
Stay ahead in your career. Get AmbitionBox app
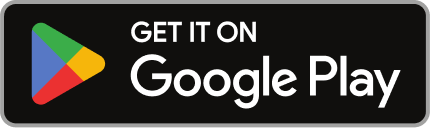
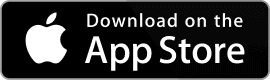
Trusted by over 1.5 Crore job seekers to find their right fit company
80 L+
Reviews
10L+
Interviews
4 Cr+
Salaries
1.5 Cr+
Users
Contribute to help millions
AmbitionBox Awards
Get AmbitionBox app
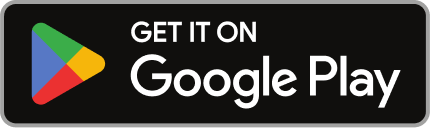
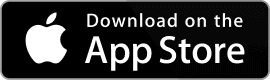