BST Iterator Problem Statement
You are tasked with implementing a class BSTIterator
, which is designed to traverse a Binary Search Tree (BST) in the inorder manner. The class must support the following operations:
BSTIterator(Node root)
- A constructor that initializes the iterator with the root of the BST.next()
- Returns the next element in the inorder traversal.hasNext()
- Returns true if there are more elements to traverse, false otherwise.prev()
- Returns the previous element from the current inorder traversal point.hasPrev()
- Returns true if there is a previous element to access, false otherwise.
Input:
The input starts with an integer 'T', representing the number of test cases. Each test case consists of a single line containing the tree's elements in level-order traversal format, with tree node values separated by spaces. Use -1 to represent null nodes.
Output:
For each test case, output a single line containing space-separated integers, which represents the inorder traversal of the binary search tree.
Example:
Input:
4
2 6
1 3 5 7
-1 -1 -1 -1 -1 -1
Output:
1 2 3 4 5 6 7
Constraints:
1 <= T <= 10
1 <= N <= 10^4
1 <= A[i] <= 10^9
Note:
While reading the tree input, the first not null node of a level becomes the parent for its left and right children in the next level. Continue this until all nodes at the last level are processed. You do not need to handle output printing as it is already managed.
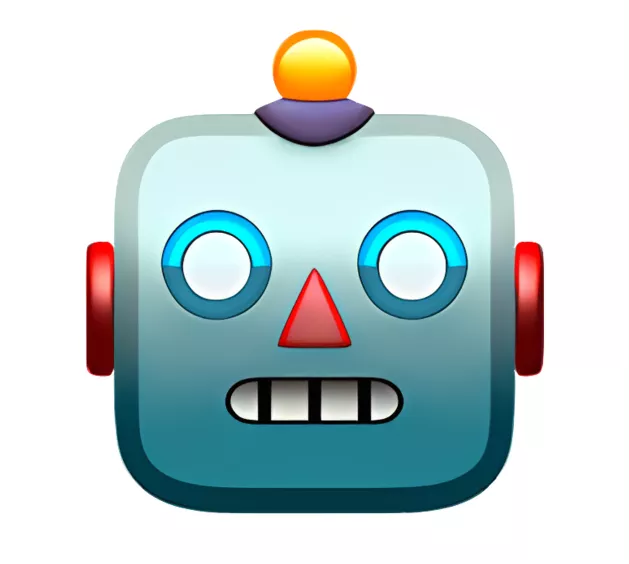
AnswerBot
4mo
Implement a BSTIterator class to traverse a Binary Search Tree in inorder manner.
Implement a constructor to initialize the iterator with the root of the BST.
Implement next() and hasNext() methods to t...read more
Help your peers!
Add answer anonymously...
Amazon Software Developer interview questions & answers
A Software Developer was asked 1mo agoQ. Could you describe the process for designing a data structure that allows for al...read more
A Software Developer was asked 1mo agoQ. What is MySQL?
A Software Developer was asked 1mo agoQ. What is Java?
Popular interview questions of Software Developer
A Software Developer was asked 1mo agoQ1. Could you describe the process for designing a data structure that allows for al...read more
A Software Developer was asked 1mo agoQ2. What is MySQL?
A Software Developer was asked 1mo agoQ3. What is Java?
Top HR questions asked in Amazon Software Developer
A Software Developer was asked 1mo agoQ1. Tell me about a time you had to get to the root cause of a problem
A Software Developer was asked 5mo agoQ2. What are the short-term and long-term goals for the team or organization?
A Software Developer was asked 5mo agoQ3. Why do you want to work at Amazon?
Stay ahead in your career. Get AmbitionBox app
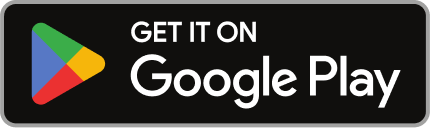
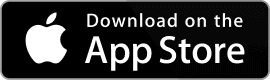
Trusted by over 1.5 Crore job seekers to find their right fit company
80 L+
Reviews
10L+
Interviews
4 Cr+
Salaries
1.5 Cr+
Users
Contribute to help millions
AmbitionBox Awards
Get AmbitionBox app
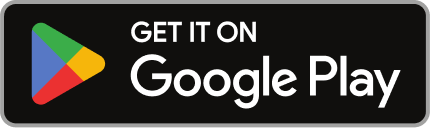
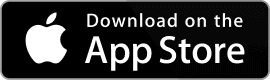