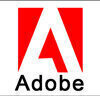
Asked in Adobe
Stack using Two Queues Problem Statement
Develop a Stack Data Structure to store integer values using two Queues internally.
Your stack implementation should provide these public functions:
Explanation:
1. Constructor: Initializes the two queue data members. 2. push(data): Accepts an integer and pushes it onto the stack. 3. pop(): Removes and returns the top element in the stack; returns -1 if empty. 4. top: Returns the top element without removing it; returns -1 if empty. 5. size(): Returns the current number of elements in the stack. 6. isEmpty(): Checks if the stack is empty and returns a boolean result.
Input:
The first line contains an integer 'Q', representing the number of queries. The next 'Q' lines specify each operation: - For push, the line contains two integers: the operation type and the integer value to push. - For other operations, the line consists of a single integer indicating the operation type.
Output:
- For Query-1, do not return anything. - For Query-2, output the popped value. - For Query-3, output the current top value. - For Query-4, output the size of the stack. - For Query-5, output 'true' or 'false'. Each query's output should be on a different line.
Example:
Input:
Q = 5 1 42 2 3 1 17
Output:
42 -1 17
Constraints:
1 <= Q <= 1000
1 <= query type <= 5
-10^9 <= data <= 10^9
and data ≠ -1
Note:
Direct output printing is handled externally. Focus on implementing the stack functionality.
Be the first one to answer
Add answer anonymously...
Top Software Quality Engineer Interview Questions Asked at Adobe
Q. Binary Tree Mirror Conversion Problem Given a binary tree, your task is to conve...read more
Q. Palindromic Partitioning Problem Statement Given a string ‘str’, calculate the m...read more
Q. Find the Second Largest Element Given an array or list of integers 'ARR', identi...read more
Interview Questions Asked to Software Quality Engineer at Other Companies
Top Skill-Based Questions for Adobe Software Quality Engineer
Data Structures Interview Questions and Answers
250 Questions
Algorithms Interview Questions and Answers
250 Questions
Stay ahead in your career. Get AmbitionBox app
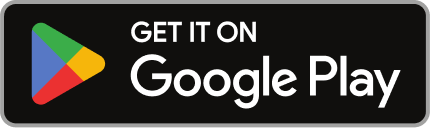
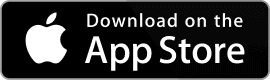
Trusted by over 1.5 Crore job seekers to find their right fit company
80 L+
Reviews
10L+
Interviews
4 Cr+
Salaries
1.5 Cr+
Users
Contribute to help millions
AmbitionBox Awards
Get AmbitionBox app
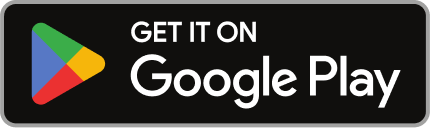
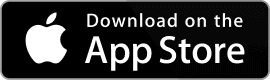