LCA of Three Nodes Problem Statement
You are given a Binary Tree with 'N' nodes where each node contains an integer value. Additionally, you have three integers, 'N1', 'N2', and 'N3'. Your task is to find the Lowest Common Ancestor (LCA) of the nodes corresponding to these three integers in the Binary Tree.
Example:
Input:
T = 1
N1 = 7, N2 = 8, N3 = 10
Binary Tree (Level Order): 1 2 3 4 -1 5 6 -1 7 -1 -1 -1 -1 -1 -1
Output:
1
Explanation:
In the given binary tree, the LCA of nodes 7, 8, and 10 is node 1.
Constraints:
- 1 <= T <= 10
- 1 <= N <= 105
- 0 <= node data <= 109
- 0 <= N1, N2, N3 <= 109
Note:
All node values in the binary tree are unique. Each of N1, N2, and N3 will always exist in the binary tree.
Input:
The first line contains an integer T, the number of test cases. For each test case:
Three integers N1, N2, and N3.
A level-order traversal of the tree nodes as space-separated integers, where -1 indicates a null node.
Output:
For each test case, return the value of the LCA node.
Note:
You do not need to print anything, just implement the given function.
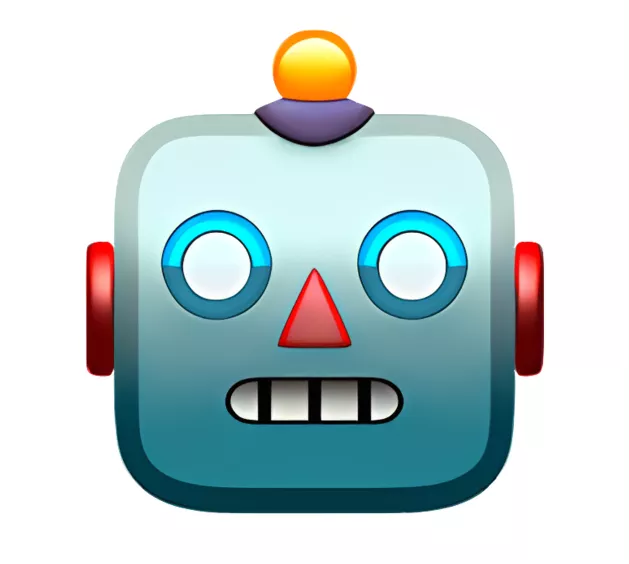
AnswerBot
4mo
Find the Lowest Common Ancestor (LCA) of three nodes in a Binary Tree.
Traverse the Binary Tree to find the paths from the root to each of the three nodes.
Compare the paths to find the common ancestor ...read more
Help your peers!
Add answer anonymously...
Stay ahead in your career. Get AmbitionBox app
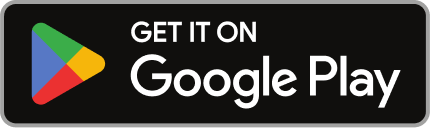
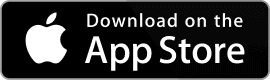
Trusted by over 1.5 Crore job seekers to find their right fit company
80 L+
Reviews
10L+
Interviews
4 Cr+
Salaries
1.5 Cr+
Users
Contribute to help millions
AmbitionBox Awards
Get AmbitionBox app
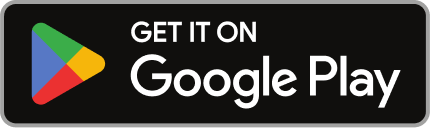
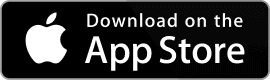